Cheat sheet and tricks for the Kotlin programming language. Used to build mobile applications.
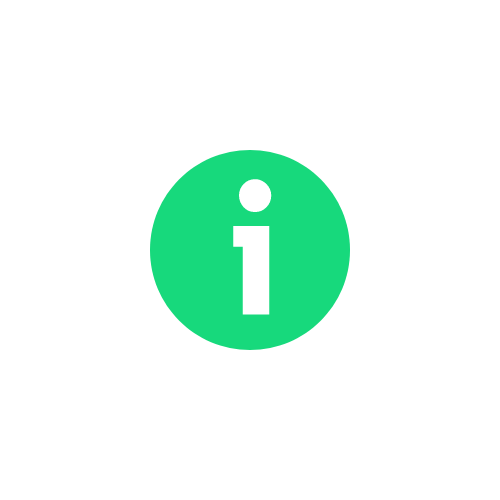
Based on Java. See Java Cheat Sheet.
Syntax
Functions
Syntax 1
fun functionName(parameter: type): returnType = functionBody
fun circumference(radius: Double): Double = 2 * pi * radius
Syntax 2
fun functionName(parameter: type): returnType { functionBody }
fun circumference(radius: Double): Double {
return 2 * pi * radius
}
Classes
Unlike Java, there is no need to define a setter and getter.
class className(var parameter: type) { functionBody}
class Circle(var radius: Double) {
private val pi: Double = 3.14
fun circumference() = 2 * pi * radius
}
val smallCircle = Circle(5.2)
Variables
val : Implicit type, null not allowed, Immutable
Like a constant.
val myName = "Nick"
var : Explicit type, null not allowed, Mutable
var myFavoriteDrink : String = "Coffee"
myFavoritDrink = "Tea" // changed the value
var + ?: Allows null, is currently null, Mutable
var whereAmI : String? = null
print("$myName drinks $myFavoriteDrink")
println(" in ${whereAmI?.uppercase() ?: "No Where"}")
Nick drinks Tea in No Where