Cheat sheet and tricks for the Java programming language.
- Java Operators (w3 schools)
Using a proxy
export _JAVA_OPTIONS="$_JAVA_OPTIONS -Djava.net.useSystemProxies=true"
Version
java -version
Java Applets
Check in the source code of the HTML page.
- Path of jar files is in parameter “codebase”.
- Search for “.jar”
- Download .jar files (URL + codebase + somefile.jar)
- Open files in a Java Decompiler like JD-GUI (see below)
Run a JAR file
java -jar filename.jar
java -cp filename.jar com.example.MainClass
Create a JAR file
jar cf jarfiletocreate.jar file-list
Open a JAR file
jar xf jar-file-to-open.jar
Compile Java code
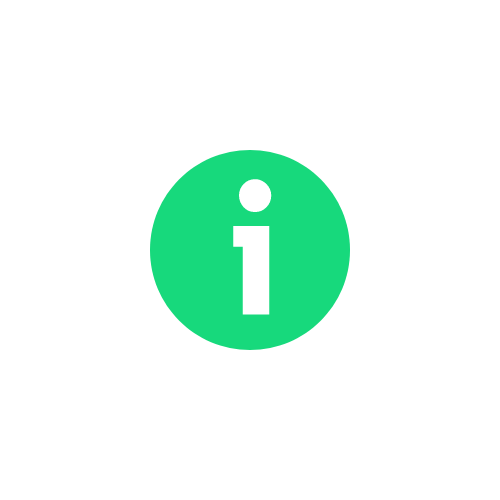
In Java, the name of the public class must match the filename. E.g. if the class is Whatever, the filename must be Whatever.java.
On Windows, download Java JDK 21 (Oracle, latest Long-Term Support (LTS) release of the Java SE Platform).
Generate Main.class
javac Main.java
Execute code
java Main
java Main "Arg 0" "Arg 1" "Arg 2" ...
Code Examples
import java.io.*;
class HelloWorld {
public static void main (String[] args) {
System.out.println("Hello World!");
}
}
Java vulnerable code
public class Main {
static void JavaInjection(String injectable) {
System.out.println("Java injection test: " + injectable);
}
public static void main(String[] args) {
JavaInjection("Test 1");
for(int i = 0; i<args.length; i++) {
System.out.println("args[" + i + "]: " + args[i]);
JavaInjection(args[i]);
}
}
}
Class Loaders
Class loaders are responsible for loading Java classes during runtime dynamically to the JVM (Java Virtual Machine) and are part of the JRE (Java Runtime Environment). The JVM does not need to know about the underlying files or file systems in order to run Java programs thanks to class loaders. These Java classes are only loaded into memory when required by the application.
Testing FAT Client Java Application
Java Decompiler
JD-GUI
JD-GUI is a standalone graphical utility that displays Java source codes of “.class” files. You can browse the reconstructed source code with the JD-GUI for instant access to methods and fields.
Installation
sudo apt install jd-gui
Start JD-GUI
Graphical interface.
jd-gui
Usage
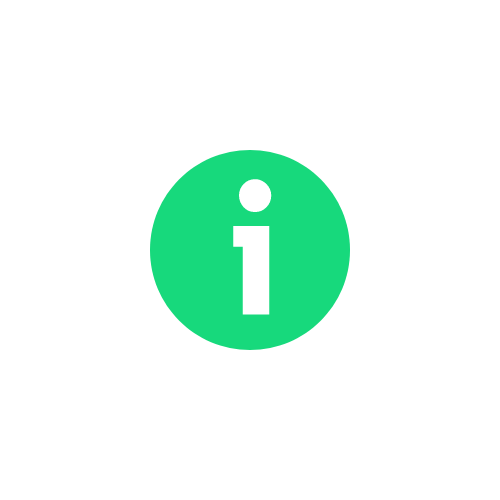
Use the Search, select “String Constant” and look for words like “passw”
## How to launch JD-GUI ?
- Double-click on _"jd-gui-x.y.z.jar"_
- Double-click on _"jd-gui.exe"_ application from Windows
- Double-click on _"JD-GUI"_ application from Mac OSX
- Execute _"java -jar jd-gui-x.y.z.jar"_ or _"java -classpath jd-gui-x.y.z.jar org.jd.gui.App"_
### Work-around for proxy
Download jar file in home
java -jar jd-gui-1.6.6.jar
JD-Eclipse
JD-Eclipse is a plug-in for the Eclipse platform. It allows you to display all the Java sources during your debugging process, even if you do not have them all.