React is a JavaScript library for building user interfaces (GUI).
- Exploiting script injection in React
- 10 React security best practices | Cheat Sheet (PDF)
- ReactJS – Architecture
Checklist
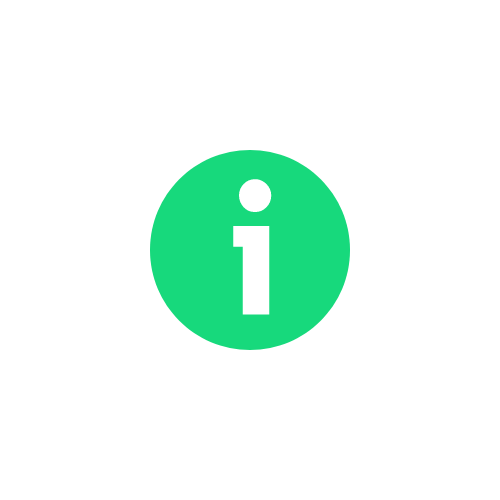
In Firefox, open the Inspector and click on the Debugger -> Sources tab to see the code of the React app.
- Frontend: look for hidden items (hidden endpoints, secrets, etc.)
- Extract all APIs enpoints from javascript to find hidden endpoints
- APIs: test as usual
- Look for “.map” at the end of compiled JS to find the original source code that is easier to read
- Search keywords like “secret”, “key”, “aes”, “authorization”, “token”, “admin”, “test”, “dev”, “stage”, “staging”, “.json”, conf/config, “POST”, “GET”, “PUT”
- Hidden flags or hidden parameters (not documented) to get other access types in the app
- Token: mechanism used to manipulate the token, roles, that is used to display the UI (User UI vs Admin UI)
- Way that HTML elements are displayed, to execute XSS (not easy by looking at the source code, easier to test dynamically)
JSX
JSX is an extension of the Javascript language. JSX prevents Injection attacks. It is safe to embed user input in JSX:
const title = response.potentiallyMaliciousInput;
// This is safe:
const element = <h1>{title}</h1>;
By default, React DOM escapes any values embedded in JSX before rendering them. Thus it ensures that you can never inject anything that’s not explicitly written in your application. Everything is converted to a string before being rendered. This helps prevent Cross Site Scripting (XSS) attacks.
URL/manifest.json
The manifest.json file is the only file that every extension using WebExtension APIs must contain. Using manifest.json, you specify basic metadata about your extension such as the name and version, and can also specify aspects of your extension’s functionality (such as background scripts, content scripts, and browser actions).
short_name: "React App"
name: "Create React App Sample"
Examples
Example 1
Run in in CodePen
function formatName(user) {
return user.firstName + ' ' + user.lastName;
}
const user = {
firstName: 'John',
lastName: 'Smith'
};
const element = (
<h1>
Hello, {formatName(user)}! </h1>
);
ReactDOM.render(
element,
document.getElementById('root')
);
Hello, John Smith!
Example 2
like_button.js
'use strict';
const e = React.createElement;
class LikeButton extends React.Component {
constructor(props) {
super(props);
this.state = { liked: false };
}
render() {
if (this.state.liked) {
return 'You liked this.';
}
return e(
'button',
{ onClick: () => this.setState({ liked: true }) },
'Like'
);
}
}
const domContainer = document.querySelector('#like_button_container');
ReactDOM.render(e(LikeButton), domContainer);
index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>Add React in One Minute</title>
</head>
<body>
<h2>Add React in One Minute</h2>
<p>This page demonstrates using React with no build tooling.</p>
<p>React is loaded as a script tag.</p>
<!-- We will put our React component inside this div. -->
<div id="like_button_container"></div>
<!-- Load React. -->
<!-- Note: when deploying, replace "development.js" with "production.min.js". -->
<script src="https://unpkg.com/react@16/umd/react.development.js" crossorigin></script>
<script src="https://unpkg.com/react-dom@16/umd/react-dom.development.js" crossorigin></script>
<!-- Load our React component. -->
<script src="like_button.js"></script>
</body>
</html>